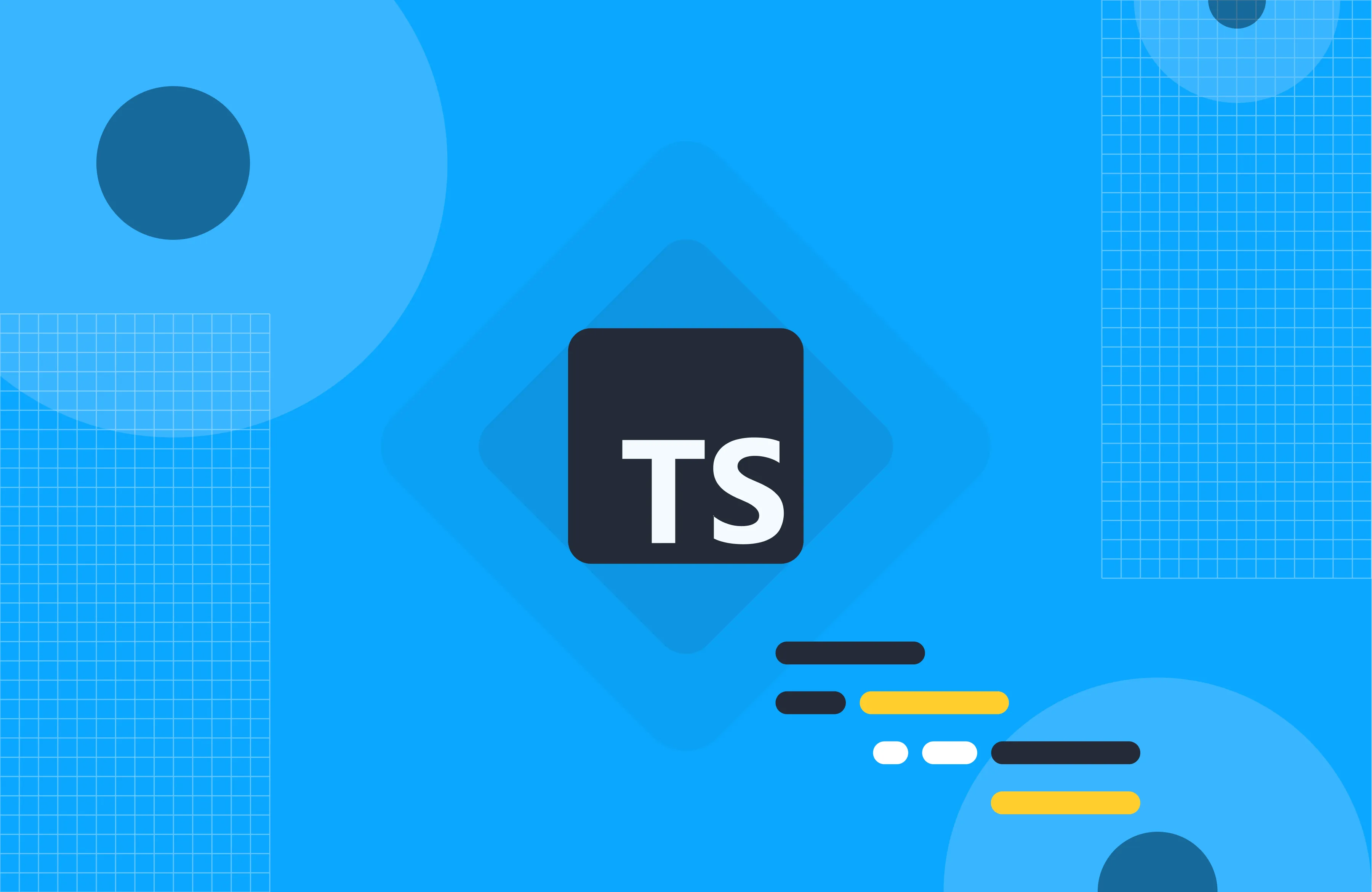
TypeScript Note
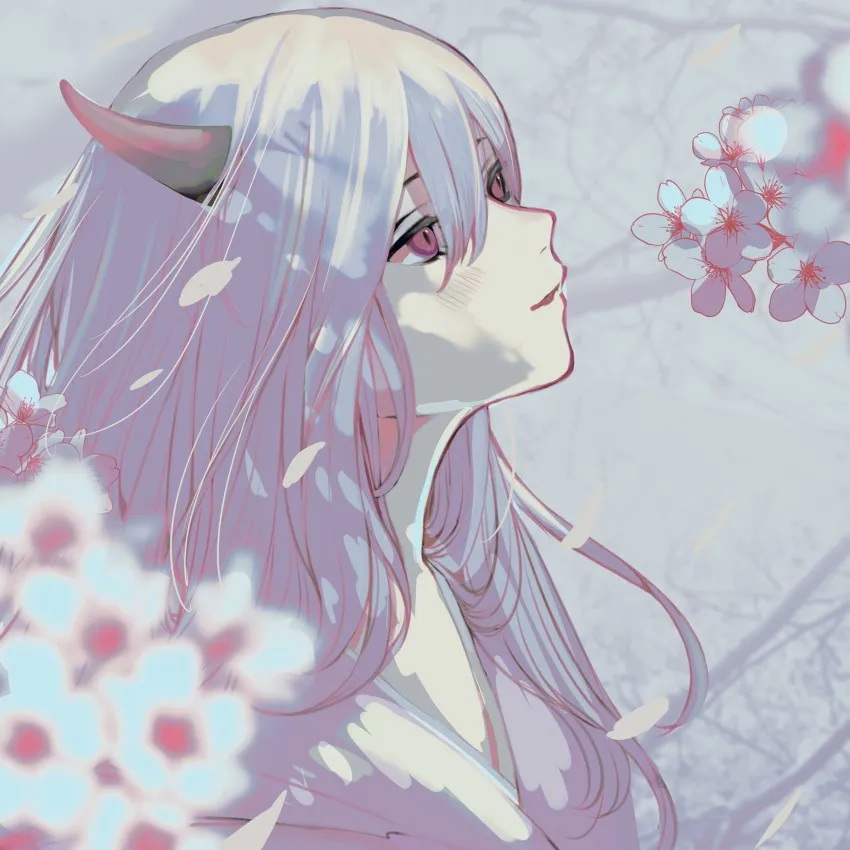
越来越像 c++ 了
它是 JavaScript 的一个超集。
经过编译后变成 JavaScript 才可以在浏览器中运行。
基础
自动识别类型
1 | let num = 1 |
变量一经赋值,它的类型也就确定下来了,直接修改会报错。
类型指定
定义时我们便可以定义它的类型。
1 | let num: number |
类型断言
1 | let numArr = [1, 2, 3] |
如果不加断言 as number
会导致错误,因为可能返回的值是 undefined
,而 undefined
是不能进行数学运算的,如果我们确定它的值类型一定,便可以添加断言,这样就不会导致报错。
在 JavaScript 中,undefined
则会被当作 NaN
进行数学运算。
基础类型
- string
- number
- boolean
- null
- undefined
- void
- 值只有
undefined
,函数的类型
- 值只有
联合类型
如果一个值可能有多个类型,定义时使用 |
隔开即可。
1 | let v: string | null = null |
null
值默认可以分配给任意类型,开启严格配置后,只可以分配给 null
类型,所以需要通过这种方式声明一下。
也可以用它来限制取值,如:
1 | let v: 1 | 2 | 3 |
这样它就只能取这三个值。
元组
1 | let t: [number, string, number?] = [1, 'a', 2] |
类似于数组,预定类型。
如果该值可选,加个问号即可。
枚举类型
1 | enum My { |
接口
1 | interface Obj { |
类型别名
1 | type Mytype = string | number |
泛型函数
1 | function fn<T>(a: T, b: T): T[] { |
进阶
函数重载
1 | function hello (name: string): string |
其实只保留最后一行函数定义也没问题,这样的好处是 vsc 会提示你函数结构。
接口继承
1 | interface Parent { |
类构造函数
1 | class Article { |
存取器
1 | class User { |
抽象类
可能不会被实例化,只是作为基类给其它类规范格式的。
1 | abstract class Animal { |
抽象类中可以有抽象属性,这样被子类继承时子类必须实现抽象属性,而非抽象属性子类可以正常继承使用。
接口实现
1 | interface Animal { |
可以继承多个接口,逗号隔开即可,但不能继承多个类。
泛型类
1 | class MyClass<T> { |
与泛型函数类似。
- Title: TypeScript Note
- Author: Falling_Sakura
- Created at : 2024-08-03 17:45:09
- Updated at : 2025-03-07 14:53:55
- Link: https://vercel.fallingsakura.top/a4a6c774.html
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments